Create a Delivery Preview for a Product Detail Page
Buy with Prime API is now available for early access
Sign up for early access to the Buy with Prime API using the 'Sign Up' button below. The API may change as Amazon receives feedback and iterates on it.
This topic contains examples of requests and responses that show how to Create Delivery Previews that you can display on a product detail page.
Delivery Preview on a Product Detail Page
You can create delivery previews for both products eligible for Buy with Prime and products that aren't eligible for Buy with Prime. You can display delivery estimates across product detail pages, the shopping cart, and checkout. The deliveryPreview
query supports all existing Amazon fulfillment delivery speeds, such as priority and standard. You can display delivery offers for Prime members as well as shoppers who aren't Prime members.
To generate a precise delivery preview that you can use to create an order, use accurate delivery terms. In the deliveryPreview
query, include the shopper identity, and in DeliveryLocationInput
include all attributes related to the shipping address.
The following example shows the response to a query to the Delivery Preview interface.
Example Delivery Preview Response
{
"data":{
"deliveryPreview":{
"id":"product-a-delivery-preview",
"deliveryGroups":[
{
"id":"product-a-delivery-group",
"products":[
{
"productIdentifier":{
"value":"product-a-id-1"
},
"amount": {
"unit:" "UNIT",
"value": 1
}
}
],
"deliveryOffers":[
{
"id":"product-a-delivery-offer-1",
"date":{
"earliest":"2024-04-01T04:00:00Z",
"latest":"2024-04-01T04:00:00Z"
},
"deliveryTerms":{
"isPrimeEligible": true
},
"policy":{
"messaging":{
"messageText":"Get it as soon as Tomorrow, April 1st",
"locale":"en-US",
"badge":"PRIME"
}
},
"expiresAt":null
},
{
"id":"product-a-delivery-offer-2",
"date":{
"earliest":"2024-04-03T04:00:00Z",
"latest":"2024-04-03T04:00:00Z"
},
"deliveryTerms":{
"isPrimeEligible": true,
"deliverySpeed":"Standard"
},
"policy":{
"messaging":{
"messageText":"Get it April 3rd",
"locale":"en-US",
"badge":"PRIME"
}
},
"expiresAt":null
}
]
}
]
}
}
}
The following checkout image shows delivery offers for a product that isn't eligible for Buy with Prime.
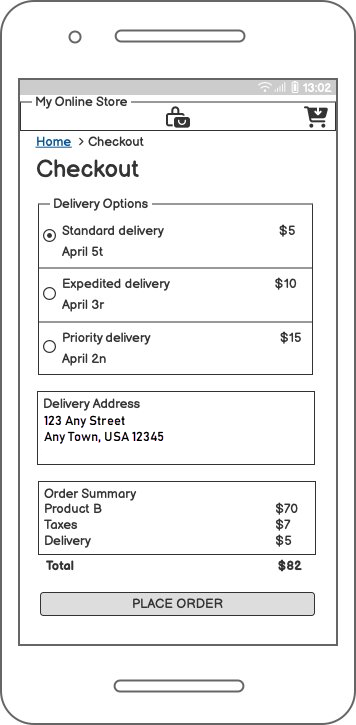
Delivery offers for a product that isn't eligible for Buy with Prime
The following checkout image shows a cart that has one product eligible for Buy with Prime, and one product that isn't eligible for Buy with Prime.
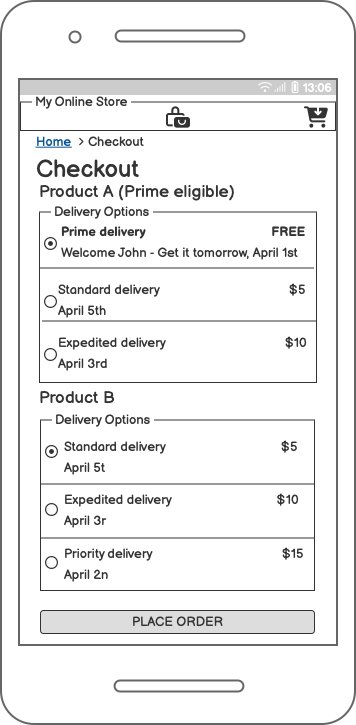
Delivery offers for a product eligible for Buy with Prime and a product that isn't eligible for Buy with Prime
The examples are ordered such that they use and display an increasing amount of shopper data to refine the delivery estimates. Shopper data can be obtained from shopper input on your site or from a shopper’s Amazon account (if they're signed in). While increasing the amount of shopper data provides a more accurate delivery estimate, it can also increase the latency of the API call.
To learn how to call the Buy with Prime API, see Call the Buy with Prime API.
In the sandbox environment, to call deliveryPreview
, shopperBwPEligibility
, or createOrder
, you must use one of the Sandbox shopper identity tokens.
Sample data
The examples in this topic use the following sample data.
Sample catalog data
val inSearchOfLostTime = {
title: "In Search of Lost Time",
isbn: "example-isbn",
isPrimeIntended: true,
sku: "example-sku",
mSku: "example-msku",
imageUrl: "https://example.com/images/example-image.jpg",
detailPageUrl: "https://www.amazon.com/dp/example-detail-page-1"
};
val prideAndPrejudice = {
title: "Pride and Prejudice",
isbn: "example-isbn-2",
isPrimeIntended: true,
sku: "example-sku-2",
mSku: "example-msku-2",
imageUrl: "https://example.com/images/example-image-2.jpg",
detailPageUrl: "https://www.amazon.com/dp/example-detail-page-2"
};
Create a delivery preview with no shopper data or delivery location information
In the simplest case, you can get a delivery offer by providing only product information, and no additional shopper or delivery details. The following query is the easiest to construct and the least accurate for a given shopper.
Request
// Variables from sample data
inSearchOfLostTime
// GraphQL query
query deliveryPreview($input: DeliveryPreviewInput!) {
deliveryPreview(input: $input) {
id
deliveryGroups {
id
deliveryOffers {
id
date {
earliest
latest
}
policy {
messaging {
messageText
locale
badge
}
}
expiresAt
}
}
}
}
// Query variables
{
"input": {
"products": [
{
"productIdentifier": {
"SKU": inSearchOfLostTime.sku
},
"amount": {
"unit:" "UNIT",
"value": 1
}
}
]
}
}
Response
{
"data":{
"deliveryPreview":{
"id":"example-delivery-preview-id",
"deliveryGroups":[
{
"id":"example-delivery-group-id",
"deliveryOffers":[
{
"id":"example-delivery-offer-id",
"date":{
"earliest":"2023-12-07T04:00:00Z",
"latest":"2023-12-07T04:00:00Z"
},
"deliveryTerms":{
"isPrimeEligible": true
},
"policy":{
"messaging":{
"messageText":"Get it as soon as Tomorrow Dec 6",
"locale":"en-US",
"badge":"PRIME"
}
},
"expiresAt":null
}
]
}
]
}
}
}
Create a delivery preview with the shopper's IP address
If you have access to the shopper's IP address, you can enhance delivery offers by using geolocation data associated with the IP address. This scenario relies on the current virtual location of the shopper and tends to be more accurate than creating a delivery preview with no shopper data.
Request
# Variables from sample data
inSearchOfLostTime
// GraphQL query
query deliveryPreview($input: DeliveryPreviewInput!) {
deliveryPreview(input: $input) {
id
deliveryGroups {
id
deliveryOffers {
id
date {
earliest
latest
}
policy {
messaging {
messageText
locale
badge
}
}
expiresAt
}
}
}
}
// Query variables
{
"input": {
"products": [
{
"productIdentifier": {
"SKU": inSearchOfLostTime.sku
},
"amount": {
"unit:" "UNIT",
"value": 1
}
}
],
"location": {
"ipAddress": "192.0.2.0",
}
}
}
Response
{
"data":{
"deliveryPreview":{
"id":"example-delivery-preview-id",
"deliveryGroups":[
{
"id":"example-delivery-group-id",
"deliveryOffers":[
{
"id":"example-delivery-offer-id",
"date":{
"earliest":"2023-12-08T04:00:00Z",
"latest":"2023-12-08T04:00:00Z"
},
"deliveryTerms":{
"isPrimeEligible": true
},
"policy":{
"messaging":{
"messageText":"Get it as soon as Thu, Dec 7",
"locale":"en-US",
"badge":"PRIME"
}
},
"expiresAt":null
}
]
}
]
}
}
}
Create a delivery preview with the shopper's postal code
If you have access to the shopper's postal code, you can refine delivery offers by using geolocation data associated with the postal code. A shopper can provide their postal code either indirectly through their signed-in identity, or directly through an experience on the product detail page, which allows postal code input. In either case, this scenario provides a highly accurate delivery estimate because it requires additional context about the shopper.
For example, if the shopper signs in with their Amazon account credentials and enters a zip code on your website, you can pass both the shopper identity and the zip code to the deliveryPreview
query so that the returned delivery preview is specific to the zip code as the delivery location.
Request
# Variables from sample data
inSearchOfLostTime
// GraphQL query
query deliveryPreview($input: DeliveryPreviewInput!) {
deliveryPreview(input: $input) {
id
deliveryGroups {
id
deliveryOffers {
id
date {
earliest
latest
}
policy {
messaging {
messageText
locale
badge
}
}
expiresAt
}
}
}
}
// Query variables
{
"input": {
"products": [
{
"productIdentifier": {
"SKU": inSearchOfLostTime.sku
},
"amount": {
"unit:" "UNIT",
"value": 1
}
}
],
"location": {
"shippingAddress": {
"postalCode": "98109"
}
}
}
}
Response
{
"data":{
"deliveryPreview":{
"id":"example-delivery-preview-id",
"deliveryGroups":[
{
"id":"example-delivery-group-id",
"deliveryOffers":[
{
"id":"example-delivery-offer-id",
"date":{
"earliest":"2023-12-07T04:00:00Z",
"latest":"2023-12-07T04:00:00Z"
},
"deliveryTerms":{
"isPrimeEligible": true
},
"policy":{
"messaging":{
"messageText":"Get it as soon as Tomorrow Dec 6",
"locale":"en-US",
"badge":"PRIME"
}
},
"expiresAt":null
}
]
}
]
}
}
}
Create a delivery preview with the shopper's delivery address
For more accurate and detailed delivery offers, you can include the shopper's complete delivery address. A shopper can provide their delivery address either indirectly through their identity (if they're signed in), or directly by choosing their delivery location on a product detail page. In either case, this scenario provides the most accurate delivery estimate because it requires the most context about the shopper.
Request
# Variables from sample data
inSearchOfLostTime
// GraphQL query
query deliveryPreview($input: DeliveryPreviewInput!) {
deliveryPreview(input: $input) {
id
deliveryGroups {
id
deliveryOffers {
id
date {
earliest
latest
}
policy {
messaging {
messageText
locale
badge
}
}
expiresAt
}
}
}
}
// Query variables
{
"input": {
"products": [
{
"productIdentifier": {
"SKU": inSearchOfLostTime.sku
},
"amount": {
"unit:" "UNIT",
"value": 1
}
}
],
"location": {
"shippingAddress": {
"name": "John Doe",
"streetAddress": "410 Terry Ave N",
"locality": "Seattle",
"region": "WA",
"postalCode": "98109",
"countryCode": "US"
}
}
}
}
Response
{
"data":{
"deliveryPreview":{
"id":"example-delivery-preview-id",
"deliveryGroups":[
{
"id":"example-delivery-group-id",
"deliveryOffers":[
{
"id":"example-delivery-offer-id",
"date":{
"earliest":"2023-12-07T04:00:00Z",
"latest":"2023-12-07T04:00:00Z"
},
"deliveryTerms":{
"isPrimeEligible": true
},
"policy":{
"messaging":{
"messageText":"Get it as soon as Tomorrow Dec 6",
"locale":"en-US",
"badge":"PRIME"
}
},
"expiresAt":null
}
]
}
]
}
}
Create a delivery preview with the shopper's Amazon identity
If the shopper is identified through their shopper identity token, the delivery preview can be tailored to display delivery offers based on their Amazon account information.
If the shopper signs in with their Amazon account credentials but provides no other delivery location preferences for you to pass to the deliveryPreview
query, the returned delivery preview is based on the default address in the shopper's address book.
Request
# Variables from sample data
inSearchOfLostTime
// GraphQL query
query deliveryPreview($input: DeliveryPreviewInput!) {
deliveryPreview(input: $input) {
id
deliveryGroups {
id
deliveryOffers {
id
date {
earliest
latest
}
policy {
messaging {
messageText
locale
badge
}
}
expiresAt
}
}
}
}
// Query variables
{
"input": {
"products": [
{
"productIdentifier": {
"SKU": inSearchOfLostTime.sku
},
"amount": {
"unit:" "UNIT",
"value": 1
}
}
],
"shopperIdentity": {
"lwaAccessToken":{
"value": "EXAMPLE_LWA_ACCESS_TOKEN",
"externalId": "EXAMPLE_EXTERNAL_ID"
}
}
}
}
Response
{
"data":{
"deliveryPreview":{
"id":"example-delivery-preview-id",
"deliveryGroups":[
{
"id":"example-delivery-group-id",
"deliveryOffers":[
{
"id":"example-delivery-offer-id",
"date":{
"earliest":"2023-12-07T04:00:00Z",
"latest":"2023-12-07T04:00:00Z"
},
"deliveryTerms":{
"isPrimeEligible": true
},
"policy":{
"messaging":{
"messageText":"Get it as soon as Tomorrow Dec 6",
"locale":"en-US",
"badge":"PRIME"
}
},
"expiresAt":null
}
]
}
]
}
}
Related topics
Updated about 2 months ago